Quickstart
Serialization
JsonDocument doc;
doc["sensor"] = "gps";
doc["time"] = 1351824120;
doc["data"][0] = 48.756080;
doc["data"][1] = 2.302038;
serializeJson(doc, Serial);
// This prints:
// {"sensor":"gps","time":1351824120,"data":[48.756080,2.302038]}
Deserialization
char json[] = "{\"sensor\":\"gps\",\"time\":1351824120,\"data\":[48.756080,2.302038]}";
JsonDocument doc;
deserializeJson(doc, json);
const char* sensor = doc["sensor"];
long time = doc["time"];
double latitude = doc["data"][0];
double longitude = doc["data"][1];
Features
Intuitive Syntax
ArduinoJson has a simple and intuitive syntax to handle objects and arrays.
Fetch data:
const char* city = doc["city"];
double temp = doc["weather"]["temp"];
Set data:
doc["city"] = "Paris";
doc["weather"]["temp"] = 21.2;
Serialize and Deserialize
ArduinoJson supports both JSON serialization and deserialization.
Read JSON:
deserializeJson(doc, myInput);
…modify:
doc["last_modification"] = time();
…write back:
serializeJson(doc, myOutput);
Input filter
ArduinoJson can filter large inputs to keep only the fields that are relevant to your application, thereby saving a lot of memory.
JsonDocument filter;
filter["list"][0]["temperature"] = true;
deserializeJson(doc, input, DeserializationOption::Filter(filter));
Parse From Stream
ArduinoJson can parse directly from an input Stream
or std::istream
Parse input from the serial port:
deserializeJson(doc, Serial);
…an Ethernet connection
deserializeJson(doc, ethernetClient);
…or a Wifi connection
deserializeJson(doc, wifiClient);
It also supports custom reader types.
Print to Stream
ArduinoJson is able to print directly to a Print
or std::ostream
Send the JSON to the serial port:
serializeJson(doc, Serial);
…an Ethernet connection
serializeJson(doc, ethernetClient);
…or a Wifi connection
serializeJson(doc, wifiClient);
It also supports custom writer types.
Indented output
ArduinoJson can produce compact:
{"ports":[80,443]}
…or prettified documents:
{
"ports": [
80,
443
]
}
Flash Strings
ArduinoJson works directly with strings stored in program memory (PROGMEM
)
You can use a Flash String as JSON input
deserializeJson(doc, F("{\"city\":\"Paris\",\"temp\":18.5}"));
…as a value
doc["cond"] = F("Sunny");
…or as a key
float temp = doc[F("temp")];
String deduplication
ArduinoJson deduplicates strings in the JSON document.
When you have several identical keys or values, the JsonDocument
only stores one of each.
In practice, this feature reduces memory consumption by 20-30%.
Implicit or Explicit Casts
ArduinoJson supports two coding styles, with implicit or explicit casts
Implicit cast:
const char* city = doc["city"];
float temp = doc["temperature"];
Explicit cast:
auto city = doc["city"].as<const char*>();
auto temp = doc["temperature"].as<float>();
Unicode
ArduinoJson can decode UTF-16 escape sequences to UTF-8.
deserializeJson(doc, "{\"name\":\"Beno\u00EEt");
const char* name = doc["name"]; // <- "Benoît"
Comments
ArduinoJson supports comments in the input document.
{
/* The WiFi configuration */
"wifi": {
"ssid": "The Bat Cave",
"pass": "imbatman" // <- not secure!!
}
}
Optional feature, see ARDUINOJSON_ENABLE_COMMENTS
NDJSON, JSON Lines
When parsing a JSON document from an input stream, ArduinoJson stops reading as soon as the document ends (e.g., at the closing brace).
This feature allows reading JSON documents one after the other; for example, you can use it to read line-delimited formats like NDJSON or JSON Lines.
{"event":"add_to_cart"}
{"event":"purchase"}
Efficient
Compared to the “official” Arduino_JSON library:
- ArduinoJson is twice smaller,
- ArduinoJson runs almost 10% faster,
- ArduinoJson consumes roughly 10% less RAM.
MessagePack
In addition to JSON, ArduinoJson supports MessagePack, an alternative serialization format.
MessagePack is like a binary version of JSON. You can switch from one format to the other with a single function call!
Reentrant
As long as you use a different JsonDocument
in each thread, there is no risk of race condition.
Overflow Safe
ArduinoJson protects you against integer overflows. Before casting a value to an integer, it checks that the value is in range.
If the value doesn’t fit, it returns 0
to prevent overflows.
doc["value"].as<int8_t>(); // 0
doc["value"].as<int16_t>(); // 20000
You can also safely test the type:
doc["value"].is<int8_t>(); // false
doc["value"].is<int16_t>(); // true
Custom converters
You can extend the types supported by ArduinoJson with custom converters.
For example, it allows you to add support for the (almost-)standard tm
structure:
struct tm timeinfo = *gmtime(&time);
// set {"time":"2021-05-04T13:13:04Z"}
doc["time"] = timeinfo;
// parse {"time":"2021-05-04T13:13:04Z"}
timeinfo = doc["time"];
Custom allocator
ArduinoJson supports custom allocators, in case you need something special, like using the external PSRAM on your ESP32:
struct SpiRamAllocator : ArduinoJson::Allocator {
void* allocate(size_t size) override {
return heap_caps_malloc(size, MALLOC_CAP_SPIRAM);
}
void deallocate(void* pointer) override {
heap_caps_free(pointer);
}
void* reallocate(void* ptr, size_t new_size) override {
return heap_caps_realloc(ptr, new_size, MALLOC_CAP_SPIRAM);
}
};
Portable
Despite its name, ArduinoJson is not limited to Arduino and can be used on any C++ project. All it requires a C++11-capable compiler, a requirement met by the major compilers since 2014.
ArduinoJson is continuously tested on:
- GCC 5, 6, 8, 9, 10, 11, and 12
- Clang 3.9, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, and 15
- Visual Studio 2017, 2019, and 2022
Support for older compilers is available up to ArduinoJson 6.20.
Self Contained
ArduinoJson doesn’t depend on any library; in fact, it doesn’t even depend on Arduino!
It means you can use ArduinoJson anywhere you can compile C++ code.
The best example is your test suite: you can comfortably test your program on your computer without touching a microcontroller.
Zero warning
ArduinoJson compiles without any warning, even with the highest compiler settings:
-Wall -Wextra -pedantic
on GCC/Clang/W4
on MSVC
It also has zero warning on Clang-Tidy.
Well tested
Because ArduinoJson is developed with TDD, it is thoroughly tested.
With more than 3600 assertions, ArduinoJson reaches a test coverage above 99%, making it the most tested Arduino library ever!
Header-only
ArduinoJson is a header-only library, meaning that all the code is in the headers.
This dramatically simplifies the compilation as you don’t have to worry about compiling and linking the library.
A single file distribution is also available; that way, you just need to download one header.
Try online
You can try ArduinoJson online on wandbox.org:
- JsonParserExample 7.0.4
- JsonGeneratorExample 7.0.4
- MsgPackParser 7.0.4
Documentation
Of all Arduino libraries, ArduinoJson is by far the one with the best documentation. On this website, you’ll find 724 pages of documentation and 43 news articles.
The site also hosts the ArduinoJson Assistant, an online tool that helps you to write the code for your project.
There is also the ArduinoJson Troubleshooter, another online tool that helps you solve common issues by asking a series of questions (there are about 300 pages in this tool).
Continuous fuzzing
Security is considered very seriously.
That is why ArduinoJson is integrated in Google’s OSS-Fuzz program.
As such, it is continuously fuzzed in search for security vulnerabilities.
MIT License
ArduinoJson is open-source and uses one of the most permissive licenses so you can use it on any project.
✔️️ Commercial use
✔️️ Modification
✔️️ Distribution
✔️️ Private use
Sponsors
ArduinoJson is thankful to its sponsors. Please give them a visit; they deserve it!
If you run a commercial project that embeds ArduinoJson, think about sponsoring the library's development: it ensures the code your products rely on stays actively maintained. It can also give your project some exposure to the makers' community.
If you are an individual user and want to support the development (or give a sign of appreciation), consider purchasing the book Mastering ArduinoJson.
They use ArduinoJson
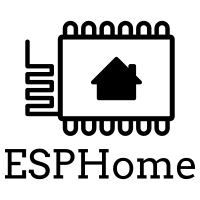
ESPHome
ESP8266 ESP32 MQTTESPHome is a system to control your ESP8266/ESP32 by simple yet powerful configuration files and control them remotely through Home Automation systems.
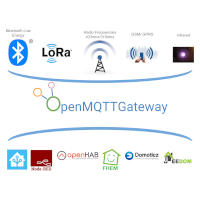
OpenMQTTGateway
ESP8266 ESP32 AVR MQTTMQTT gateway for ESP8266, ESP32, Sonoff RF Bridge or Arduino with bidirectional 433mhz/315mhz/868mhz, Infrared communications, BLE, beacons detection, mi flora, mi jia, LYWSD02, LYWSD03MMC, Mi Scale compatibility, SMS & LORA.
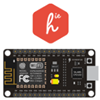
Homie for ESP8266
ESP8266 MQTTAn Arduino for ESP8266 implementation of Homie, an MQTT convention for the IoT.
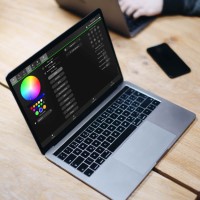
WLED
ESP32 ESP8266Control WS2812B and many more types of digital RGB LEDs with an ESP8266 or ESP32 over WiFi!
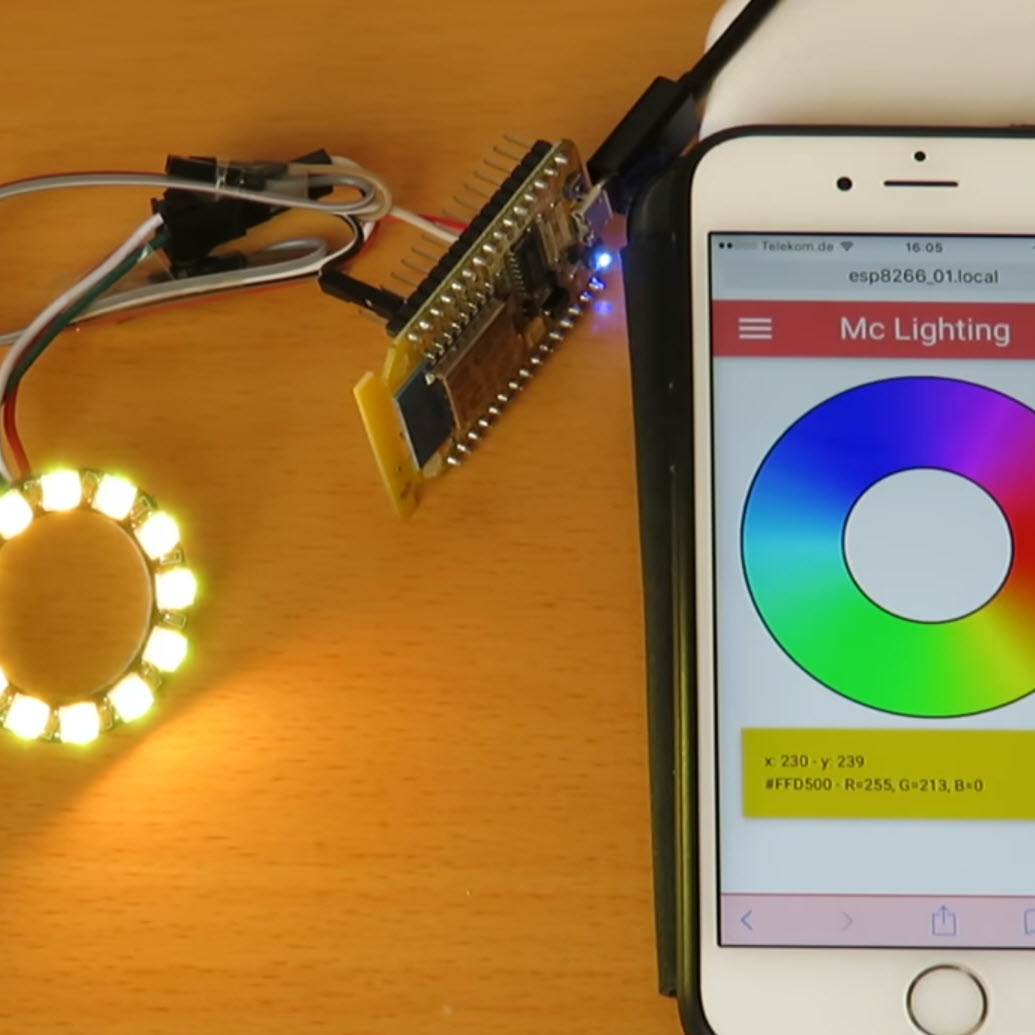
McLighting
ESP8266A very cheap internet-controllable lighting solution based on the famous ESP8266 microcontroller and WS2811/2812 led strips.
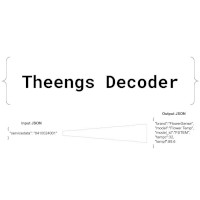
Theengs Decoder
ESP8266 ESP32Efficient, portable and lightweight library for Internet of Things messages decoding.
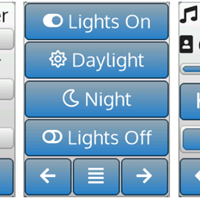
HASP - Open SwitchPlate
ESP8266 ESP32 STM32 MQTTHomeAutomation Switchplate based on lvgl for STM32F407, ESP32 & ESP8266
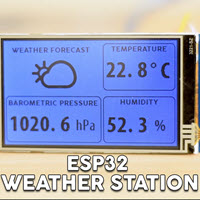
WiFi Weather Station
ESP32 OpenWeatherMapA Wifi weather station, using an ESP32 and a BME280 sensor. It fetches weather forecast from OpenWeatherMap.
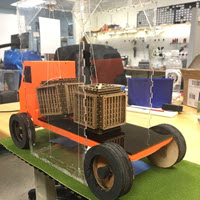
Animated truck that shows when a shuttle arrives
Particle Photon TransLoc APIThe crazy project of an animated truck that shows when a shuttle arrives.
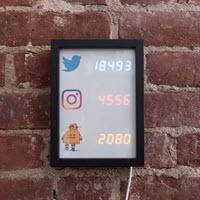
Social Stats Display
ESP8266A frame with 7-segments displays that shows the number of followers on Twitter, Instagram and Instructables.
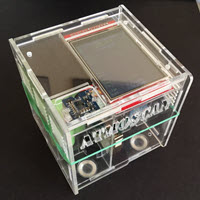
Air quality monitor
ESP8266 MQTTAtmoscan, a multisensor device aimed at monitoring indoor air quality.
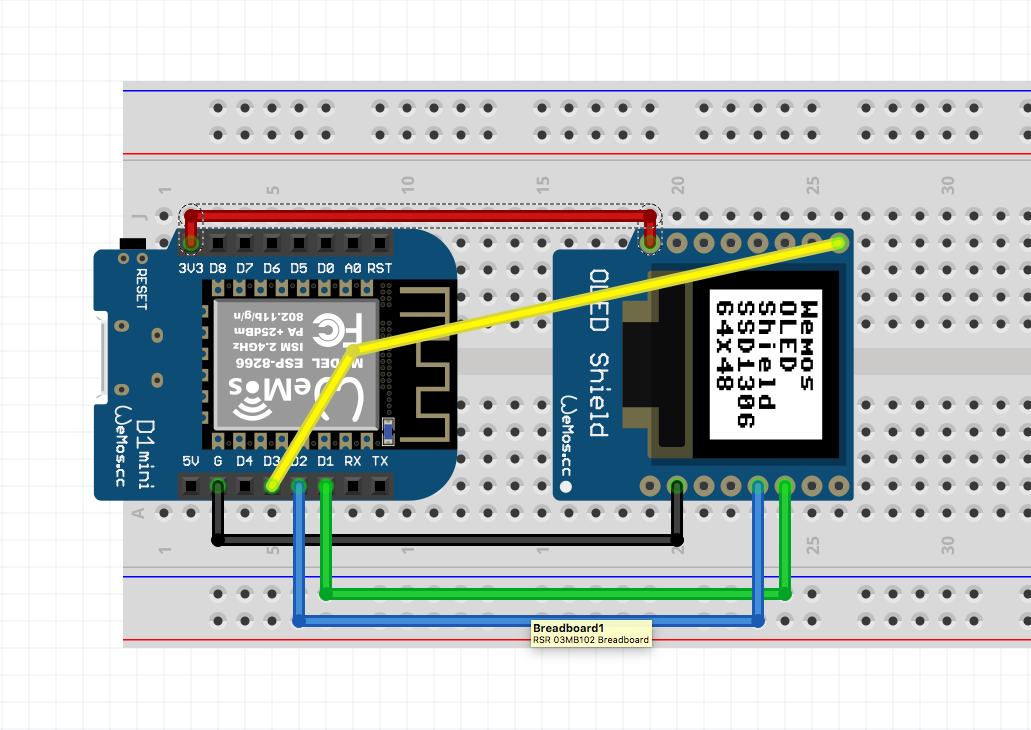
Internet clock
WeMos D1 Mini ip-api.com timezonedb.comAutoClock, a simple internet-enabled clock driven by NTP and GeoIP to give an automatic offset from UTP for your location.
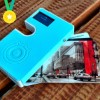
IoT Smart Wallet
ESP32 Google SpreadsheetA smart wallet with a screen to monitor cryptocurrency assets.
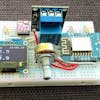
Smart(er) Underfloor Heating System
WeMos D1 MiniA thermostat that measures temperature and transfers the values to a backend. The backend analyses these values and sends instructions back to the device.
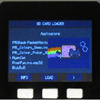
M5Stack-SD-Updater
ESP32A library for M5Stack to package you apps on a SD card and load them from a menu.
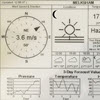
e-Paper Weather Display
ESP32 Weather UndergroundAn ESP32 and a 2.9” ePaper Display reads Weather Underground and displays the weather
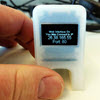
3D Printer Monitor for OctoPrint
WeMos D1 MiniMonitor your 3D Printer’s OctoPrint Server on an OLED display over a wifi connection.
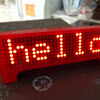
Clock News Weather Scrolling Marquee
WeMos D1 Mini coindesk.com geonames.org openweathermap.orgA LED display that shows weather, time, and many other things.
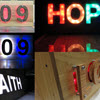
WiFi LED Light Strip Controller
NodeMCUA fully-functioning WiFi controller for your LED lights, along with an app for your iOS or Android phone or tablet.
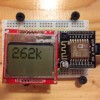
YouTube Subscriber Counter
NodeMCU YouTubeAn LCD display that shows the number of subscriber of your YouTube channel
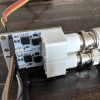
Pool Monitoring
ESP32 ThingsBoardShows pH, ORP, and temperature of a pool or spa, and uploads the data to ThingsBoard.io
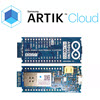
Arduino MKR1000 - DHT - Artik cloud
MKR1000 Artik CloudSending temperature and humidity from Arduino MKR1000 to Artik Cloud
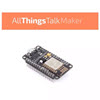
AllThingsTalk Maker Platform & NodeMCU
NodeMCU ATT MakerGetting started project for “AllThingsTalk MAKER”. Client app works on NodeMCU
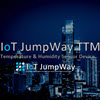
IoT JumpWay TTM ESP8266 Arduino Temperature Device
ESP8266 IoT JumpWayA temp & humidity sensing device created on an ESP8266 12F that uses the IoT JumpWay for device-to-device communication
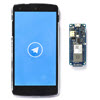
Telegram Bot Library
MKR1000 TelegramHost a Telegram Bot on your Arduino and chat with your brand new IoT device!
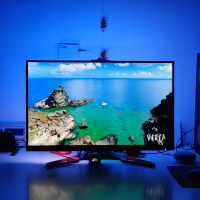
Glow Worm Luciferin
ESP32 ESP8266Bias Lighting and Ambient Light firmware, designed for Firefly Luciferin
Testimonials

Arduino makers can use ArduinoJson in their projects to connect multiple Arduino gadgets, or Arduinos to their web services. The library includes a powerful parser which can deal with nested objects (great for customising the messaging format to fit with your objectives), it is light on memory (both RAM and flash), and it has a really nice API so it’s easy to use.
Thanks for your great work on this awesome library, I will definitely keep using it in my projects and recommend to others :)
ArduinoJson library should be used as a benchmark/reference for making libraries. Truly elegant.
You’ve clearly done a tremendous amount of very good work. In fact, the existence of ArduinoJson was a key input into the decision and design process for our system’s architecture a couple years ago.
It has a really elegant, simple API and it works like a charm on embedded and Windows/Linux platforms. We recently started using this on an embedded project and I can vouch for its quality.
thegreendroid
on StackOverflow
I’ve been watching you consistently develop this library over the past six months, and I used it today for a publish and subscribe architecture designed to help hobbyists move into more advanced robotics. Your library allowed me to implement remote subscription in order to facilitate multi-processor robots.
This is a great library and I wouldn’t be able to do the project I’m doing without it. I completely recommend it.
I am just starting an ESP8266 clock project and now I can output JSON from my server script and interpret it painlessly.
I tried aJson and json-arduino before trying your library. I always ran into memory problem after a while. I have no such problem so far with your library. It is working perfectly with my web services. Thanks Benoit for a very well polished product!
That I can work through use of ArduinoJson without ever touching a MCU is a huge plus! Kudos on what must have been a non-trivial task!
Your library has greatly simplified my parsing and will make maintaining my code vastly easier in the future. I’ll certainly be buying your book to shorten my learning curve and begin to take full advantage of your knowledge and library.
It’s a great library and I have found it to be very useful in my Arduino projects. Wanted to test it on Raspberry Pi as well. Included the Headers in my project and it works flawlessly. Keep up the good work!!
ArduinoJson is for me the best json library for microcontrollers! I’m a huge fan of it!
Thanks for creating this library and supporting material - it’s incredibly well written and useful, and I appreciate it.
Richard Lyon
My son and I are working on computer game using the Unreal engine, and while the engine has JSON support, their implementation is clunky and slow. I have decided I am going to use ArduinoJSON as my JSON engine in the C++ code for the game. I am also developing a computer system for my 1966 Mustang using an ESP32 board, and I’m going to use AJSON as both the data structure to hold the car’s current status info and to send REST API calls to the Adafruit.IO IOT service. Mostly what I like best is the focus on careful memory usage. Your article about heap fragmentation is very well done, and that is what sold me on using your library.
Morris Lewis
Thanks for your great work on this library. I experimented with the hornbill library as well, it also works but I prefer yours because you’re using more of the native esp32-arduino libraries, and there’s activity on this library.
I still like ArdunioJson over the alternatives, with the fact that it will never fragment malloc memory being a clear winning advantage. Careful consideration of ArduinoJson’s limitations should allow me to use it for what I want without disadvantage.
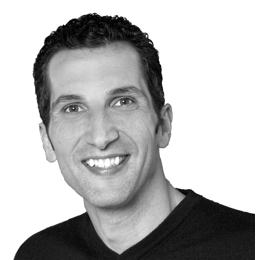
I recently stumbled onto your library after dealing with JSON on my Particle projects. Fantastic job!
Georges Auberger
Thanks very much for the lib: I have been trying to squeeze an ethernet data collector onto a nano, and I was really struggling with memory. Your implementation of json seems magic in its memory useage! What makes it stand out is the simplicity of use, and the excellent documentation.
Dr Hugh Potts - Glasgow University
Keep up the good work with
ArduinoJson
! It is an awesome library and a kind of ”beacon” for me, really. The documentation, the website in general and your book is exemplary, I love it! I also provide and maintain several open source software projects (mostly Python packages or SailfishOS apps) and try to document them as good as possible, I know how time-consuming and hard it can be.
Fantastic library. I use it not only for embedded software but also for server stuff.
Carlos Cardeñoso
ArduinoJSON does bring the best features of many such json libraries into one umbrella. Thank you for an awesome library!! :-)
Thank you again for your marvellous library which due to your book is probably one of the best documented libraries that exist. Please continue this excellent service that you provide to so many by your strive to improve this library still further and by keeping it up to date.
Dieter Grientschnig

Just wanted to say thank you for writing these docs so well. These are probably one of the easiest to understand well written docs I’ve ever browsed :)
Brad Matias
This is a fantastic library and the eBook has been a huge game changer for me with respect to optimizing the use of this library in my projects, so thanks 👍.
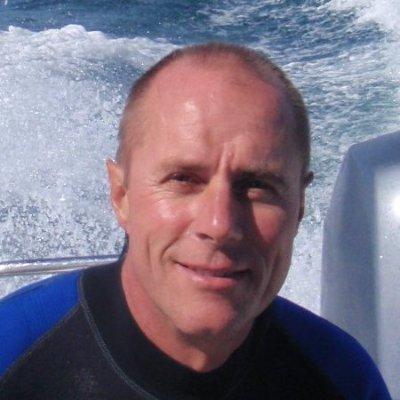
Just to send my appreciation for the good work and congratulate Benoit et al for the quality of the book “Mastering ArduinoJson”, a very good value.
Jean-Marie Locci
With regards to the documentation I don’t think I’ve seen any non-commercial library that compares in terms of documentation so I think you’re doing well! Compared to your documentation stuff like the Linus kernel is incomprehensible! Thanks again for a great library!
Just want to say that this is an impressive piece of coding. I noticed code I have never seen before, pushing the platform to it’s limits. Other languages could learn from this, complex on the inside, but how simple it is to use. Very nice :-)
ArduinoJson is a good example for great engineering. If you rely heavily on JSON, then ArduinoJson will not only give you the tools to handle data processing efficiently, but it will also be a framework to build better products upon.